TDD: The Key to Clean Code in Laravel
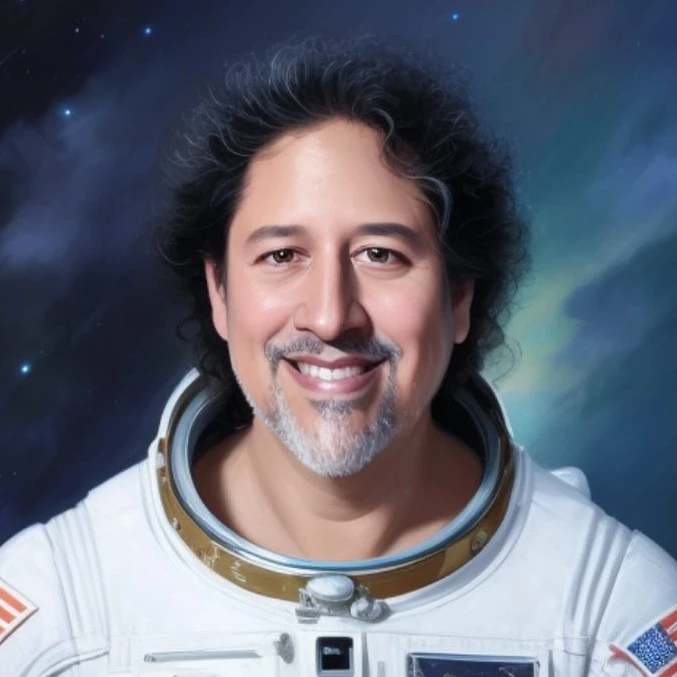
Herminio Heredia
6 months ago
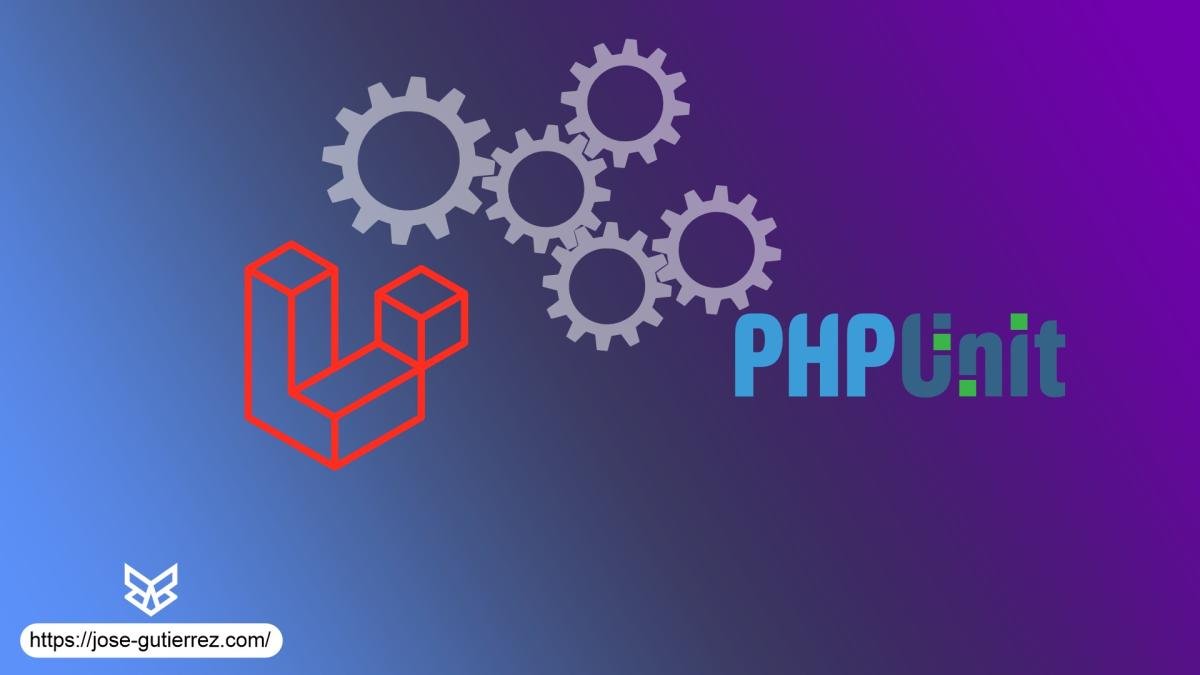
Introduction
Testing-driven development (TDD) is a foundational approach in software development that prioritizes creating reliable, clean, and maintainable code. By writing tests before implementing functionality, developers ensure that each component of an application works as intended, allowing for early detection and resolution of issues. In Laravel, TDD is a highly effective practice that helps streamline development, making it easier to build robust applications with confidence.
Imagine you're building a house. Would you start putting up walls without a solid foundation? Of course not! The same applies to software development. If you want to build a robust, maintainable, and error-free application, you need a solid foundation: tests.
Test-Driven Development (TDD) is like an architect's blueprint, a step-by-step guide that allows you to build your application with confidence, knowing that each component works correctly before adding the next.
Why TDD is Your Best Ally in Laravel
Think of TDD as your safety net. It lets you catch errors early, preventing them from becoming bigger issues later. Moreover, it helps you:
- Write Better Code: TDD forces you to think about your code's design before writing it, resulting in cleaner, modular, and easier-to-understand code.
- Save Time and Money: Detecting and fixing errors early in development is far more cost-effective than doing so in later stages.
- Refactor with Confidence: With a solid set of tests, you can refactor your code without fear of breaking existing functionality.
- Document Your Code: Tests serve as living documentation, showing how each component is expected to function.
Let’s Get Started! Building a User with TDD
Let’s look at a practical example of how to apply TDD in Laravel by creating a new user.
1. Write the Test (Red)
Start by writing a test that will fail. This ensures that you are testing the correct behavior.
<?php
namespace Tests\Feature;
use App\Models\User;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class UserTest extends TestCase
{
use RefreshDatabase;
public function test_a_user_can_be_created()
{
$userData = [
'name' => 'John Doe',
'email' => 'johndoe@example.com',
'password' => 'password123'
];
$response = $this->post('/register', $userData);
$response->assertStatus(201);
$this->assertDatabaseHas('users', [
'name' => 'John Doe',
'email' => 'johndoe@example.com',
]);
}
}
In this test, we’re simulating a POST request to the /register
route with user data. We expect the response to have a 201 status code (created) and the user to be saved in the database.
Why This Test?
It ensures that the main functionality for user registration works correctly. We validate that the controller, model, and database interact as expected.
2. Write the Minimum Code to Pass the Test (Green)
Now, write the minimum code needed to make the test pass.
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Hash;
class RegistrationController extends Controller
{
public function store(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8',
]);
$user = User::create([
'name' => $validatedData['name'],
'email' => $validatedData['email'],
'password' => Hash::make($validatedData['password']),
]);
return response()->json($user, 201);
}
}
Here, we create a controller that validates the input data, creates a new user, and saves it to the database.
3. Refactor (Refactor)
Once the test passes, we can refactor the code to improve it. In this example, we might extract the validation logic into a form request to keep the controller cleaner.
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class RegisterUserRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*/
public function authorize(): bool
{
return true;
}
/**
* Get the validation rules that apply to the request.
*/
public function rules(): array
{
return [
'name' => 'required|string|max:255',
'email' => 'required|string|email|max:255|unique:users',
'password' => 'required|string|min:8',
];
}
}
Then we modify the controller to use this new request:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use App\Http\Requests\RegisterUserRequest;
use Illuminate\Support\Facades\Hash;
class RegistrationController extends Controller
{
public function store(RegisterUserRequest $request)
{
$user = User::create([
'name' => $request->name,
'email' => $request->email,
'password' => Hash::make($request->password),
]);
return response()->json($user, 201);
}
}
And that’s it! We’ve created a user registration feature with TDD.
Benefits You'll Notice with TDD
At first, TDD may seem like it takes more time. However, as you practice, you’ll notice the following benefits:
- Increased Confidence in Your Code: You’ll know that each part of your application works as expected.
- Less Debugging Time: You’ll catch errors early, making them easier to fix.
- More Modular and Maintainable Code: TDD guides you towards cleaner and more organized design.
- Greater Productivity in the Long Run: Though it may feel slower at first, TDD will save you time and headaches down the road.
Tips for Getting Started with TDD in Laravel
- Start with Simple Tests: Don’t overcomplicate things at the beginning. Start with unit tests that verify your model and controller logic.
- Use Laravel’s Built-in Tools: Laravel includes PHPUnit and facilities for writing feature tests—take advantage of them.
- Practice, Practice, Practice: The key to mastering TDD is practice. Start with small projects and gradually increase complexity.
- Don’t Be Afraid to Ask for Help: If you encounter difficulties, don’t hesitate to seek help from the Laravel community.
Build Solid Applications with TDD!
Test-Driven Development is a powerful tool that will help you build high-quality Laravel applications. Give it a try and experience the benefits for yourself!