Taming Laziness: Lazy Objects in PHP! 💤
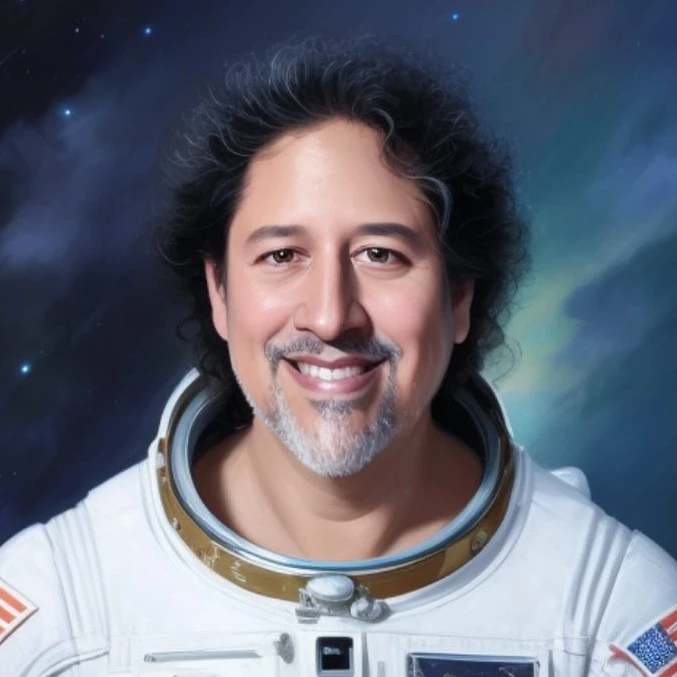
Herminio Heredia
6 months ago
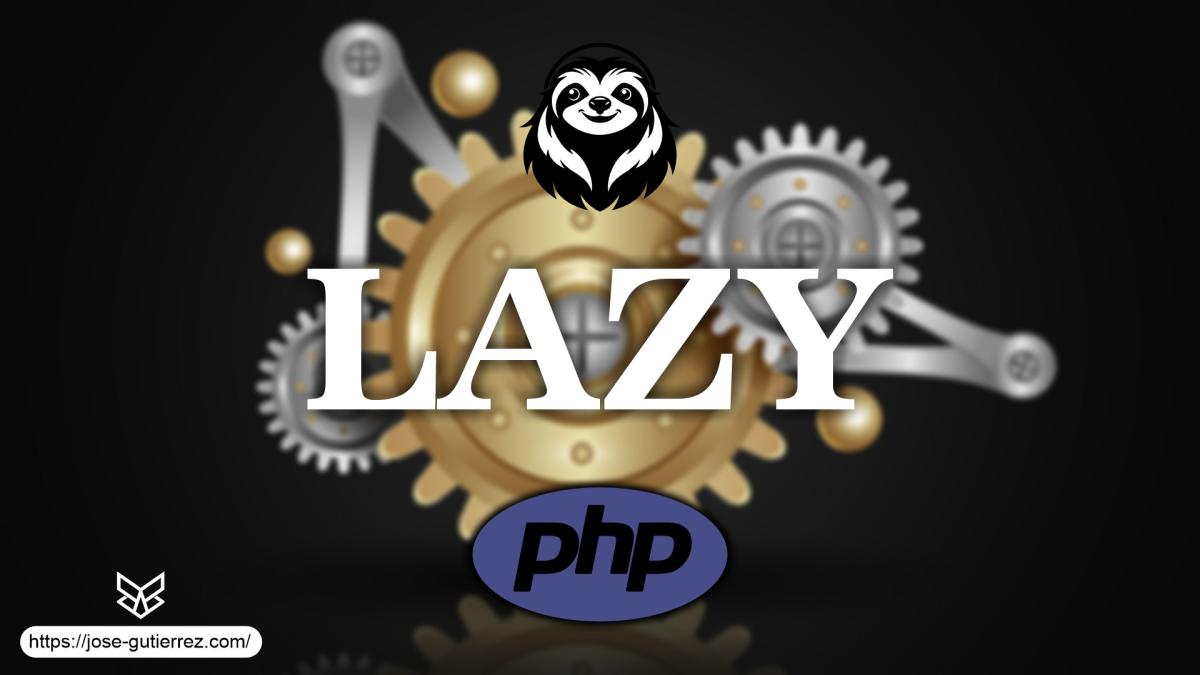
Introduction: Awaken the Power of Laziness!
In the world of web development, efficiency is key. Every millisecond counts, and optimizing our applications’ performance is a constant task. Can you imagine loading only the resources you truly need, right at the precise moment you need them? That’s exactly what lazy objects in PHP allow us to do!
Imagine you’re building an application that handles large amounts of data. Using lazy objects lets you postpone loading information until it’s strictly necessary. This can make a big difference in your application’s load speed and resource consumption.
In this post, we’ll dive deep into the concept of lazy objects in PHP. We’ll see how they work, when to use them, and how to implement them with concrete examples. Get ready to take your development skills to the next level!
What Are Lazy Objects? 🤔
A lazy object is like a student who leaves everything until the last minute. Instead of being initialized with all its data from the start, it waits until the data is genuinely needed.
Think of an ORM (Object-Relational Mapper) like Laravel’s Eloquent. When querying the database, instead of eagerly loading all the model’s relationships, we can use lazy objects so that the relationships only load when accessed. This can save a significant amount of time and resources!
PHP offers two strategies for implementing lazy objects:
- Ghost Objects: They’re like ghosts that take shape when needed. They’re initialized in the same place where they’re declared, but only when you access their properties or methods.
- Virtual Proxies: They’re like middlemen that delegate responsibility to a real object. When you access the proxy, it creates the real object and redirects all operations to it.
Both strategies have advantages and disadvantages, and choosing one depends on the specific needs of your application.
Creating Lazy Objects: Let’s Get to Work! 👷♂️
To create lazy objects in PHP, we’ll use the ReflectionClass
and ReflectionProperty
classes. Don’t worry; it’s not as complicated as it sounds!
Example 1: A Ghost in Action
class User
{
public function __construct(public string $name, public string $email)
{
echo "User created: {$this->name}\n";
}
}
$reflector = new ReflectionClass(User::class);
$lazyUser = $reflector->newLazyGhost(function (User $user) {
// Initialize the object in place
$user->__construct('Juan', 'juan@example.com');
});
var_dump($lazyUser); // The object is not initialized yet
var_dump($lazyUser->name); // It initializes when accessing the property!
In this example, the $lazyUser
object is a ghost object. By accessing the name
property, the initialization function runs, and the object takes shape.
Example 2: The Power of the Proxy
class Product
{
public function __construct(public string $name, public float $price)
{
echo "Product created: {$this->name}\n";
}
}
$reflector = new ReflectionClass(Product::class);
$lazyProduct = $reflector->newLazyProxy(function (Product $product) {
// Create and return the real instance
return new Product('Laptop', 1200.00);
});
var_dump($lazyProduct); // The object is not initialized yet
var_dump($lazyProduct->price); // It initializes when accessing the property!
Here, $lazyProduct
is a virtual proxy. When accessing the price
property, the proxy creates a real Product
object and returns its property value.
Controlling Laziness: Selective Initialization 🎛️
Sometimes, we need to control which properties trigger the initialization of a lazy object. We can do this using the skipLazyInitialization()
and setRawValueWithoutLazyInitialization()
methods of the ReflectionProperty
class.
Example 3: A Lazy yet Selective Object
class Post
{
public function __construct(public int $id, public string $title, public string $content)
{
echo "Article created: {$this->title}\n";
}
}
$reflector = new ReflectionClass(Post::class);
$lazyPost = $reflector->newLazyGhost(function ($post) {
$data = getPostData($post->id);
$post->__construct($data['id'], $data['title'], $data['content']);
});
// Prevent initialization from being triggered when accessing the 'id' property
$reflector->getProperty('id')->skipLazyInitialization($lazyPost);
$reflector->getProperty('id')->setValue($lazyPost, 123);
var_dump($lazyPost->id); // Access 'id' without initializing the object
var_dump($lazyPost->title); // Initializes upon accessing 'title'!
In this example, the id
property does not trigger the object’s initialization, whereas the title
property does.
Ghost vs. Proxy: Which One Should You Choose? 👻 vs 🎭
Both lazy object strategies have their own characteristics. Let’s look at the key differences:
-
Ghost Objects:
- They initialize in place.
- They are indistinguishable from non-lazy objects once initialized.
- Ideal when you control the instantiation and initialization of the object.
-
Virtual Proxies:
- Delegate creation of the real object to a factory function.
- Useful when you don’t control the instantiation of the real object.
- Be cautious when using their identity, because the proxy and the real object are different.
Lifecycle of a Lazy Object: From Slumber to Awakening ⏳
A lazy object can go through different stages in its lifecycle:
-
Creation: The lazy object is created using
newLazyGhost()
ornewLazyProxy()
. - Initialization: The object initializes when you access its properties or methods, or when calling specific methods to force initialization.
- Usage: Once initialized, the object behaves like any other object.
Initialization Triggers: Let the Action Begin! 🎬
Lazy objects initialize automatically when interacted with in certain ways:
- Reading or writing a property.
- Checking if a property is defined.
- Using
ReflectionProperty
to access or modify a property. - Iterating over the object’s properties with
foreach
. - Serializing the object with
serialize()
orjson_encode()
. - Cloning the object.
It’s important to note that method calls that do not access the object’s state do not trigger initialization.
Operations that Don’t Wake the Lazy Object: Shhh! 🤫
Some operations let you interact with a lazy object without initializing it:
- Using
skipLazyInitialization()
orsetRawValueWithoutLazyInitialization()
to access properties. - Getting the internal representation of properties with
get_mangled_object_vars()
. - Using
serialize()
with theReflectionClass::SKIP_INITIALIZATION_ON_SERIALIZE
option. - Calling
ReflectionObject::__toString()
. - Using
var_dump()
ordebug_zval_dump()
, unless__debugInfo()
triggers the initialization.
Initialization Sequence: Step by Step 👣
Let’s look at how a lazy object initializes, depending on the strategy used:
Ghost Objects:
- The object is marked as non-lazy.
- Uninitialized properties are set to their default values.
- The initialization function is called.
- The object is no longer lazy.
Proxy Objects:
- The object is marked as non-lazy.
- The factory function is called to create the real object.
- The proxy’s property values are discarded.
- The proxy redirects operations to the real object.
Cloning and Destruction: The Cycle of Life 🧬
When cloning a lazy object, it initializes before creating the clone. In the case of proxies, both the proxy and the real object are cloned.
The destructor of a ghost object is only called if the object has been initialized. For proxies, the destructor is called only on the real object.
Conclusion: Laziness Well-Applied! 😴
Lazy objects are a powerful tool for optimizing the performance of our PHP applications. They let us delay resource loading until it’s needed, which can improve load speed and reduce memory usage.
Remember that choosing between ghost objects and virtual proxies depends on your application’s specific needs. Experiment with both strategies and discover which one suits your case best!
I hope this post was helpful! If you’d like to stay updated on our content, remember to subscribe to our notifications.
And don’t forget to share this post with your developer friends! 😉