Managing Pivot Data in Laravel with Eloquent
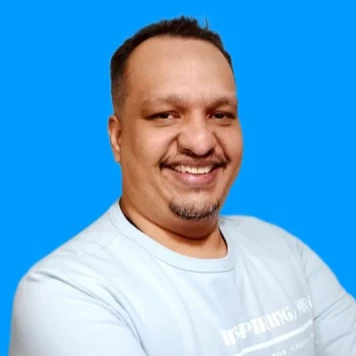
José Rafael Gutierrez
9 months ago
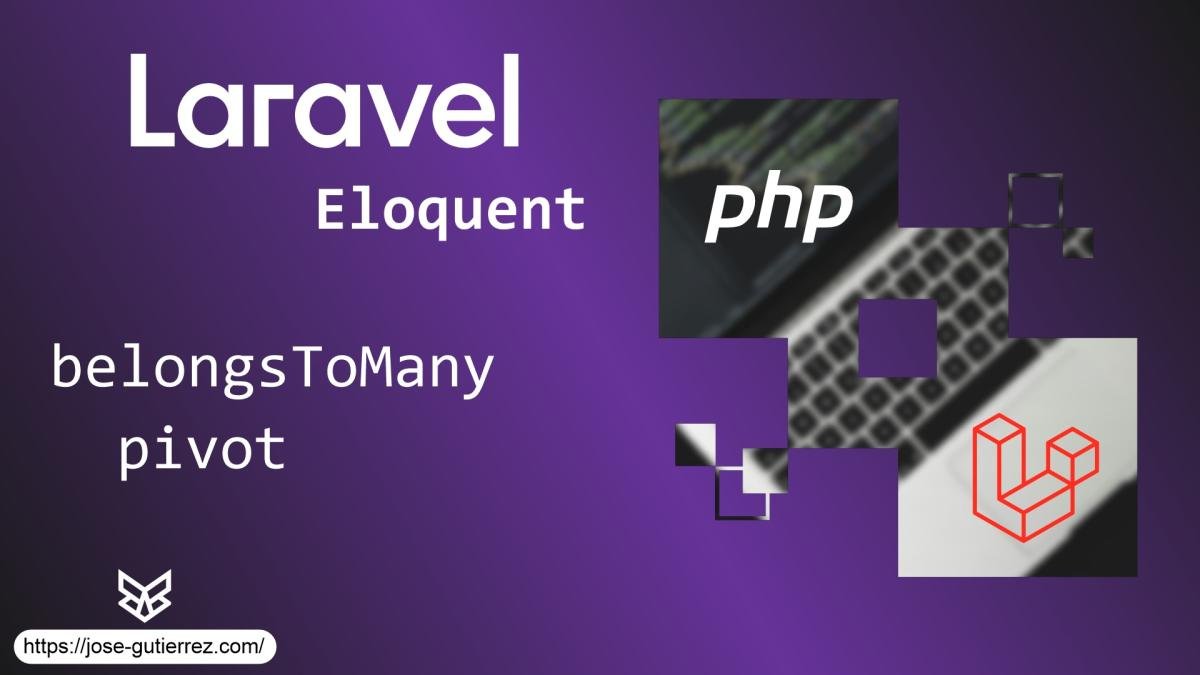
Introduction
In many-to-many relationships in Laravel, pivot tables play a crucial role. Often, aside from storing relationships, we need to add extra data, like the start date of a relationship or a user's role in a project. In this article, we will explore how to manage this data efficiently using Eloquent, with key recommendations for robust handling.
Many-to-Many Relationships in Laravel
Let's assume we have two models: User
and Project
. A user can be in multiple projects, and a project can have multiple users. This relationship is defined using the belongsToMany
method.
Basic relationship definition:
class User extends Model
{
public function projects()
{
return $this->belongsToMany(Project::class)->withPivot('role', 'start_date');
}
}
class Project extends Model
{
public function users()
{
return $this->belongsToMany(User::class)->withPivot('role', 'start_date');
}
}
The withPivot()
function is useful for accessing additional fields in the pivot table, such as role
and start_date
, without directly relying on $project->pivot
.
Adding Extra Data to the Pivot Table
If we want to add data like the user's role within a project or the start date of that relationship, we first need to modify the pivot table.
Example of a pivot table with additional data:
Schema::create('project_user', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained();
$table->foreignId('project_id')->constrained();
$table->string('role');
$table->timestamp('start_date');
});
Now, every time we associate a user with a project, we can store this additional data.
Synchronizing Relationships
One of the most common ways to manage pivot relationships is through the sync()
method. This method completely replaces any existing pivot data for the model it syncs with, which can be dangerous if not used carefully.
Example of sync()
usage:
$user->projects()->sync([
$projectId => ['role' => 'admin', 'start_date' => now()]
]);
Difference between sync()
and attach()
: It is crucial to note that sync()
removes any existing relationships not included in the sync process, while attach()
simply adds new relationships without affecting the existing ones.
Example of attach()
:
$user->projects()->attach($projectId, ['role' => 'editor', 'start_date' => now()]);
Exception Handling
It is crucial to handle errors properly to prevent code failure, for example, if projectId
does not exist.
try {
$user->projects()->attach($projectId, ['role' => 'editor', 'start_date' => now()]);
} catch (\Exception $e) {
// Handle the error, perhaps log it or display an error message
}
This improves the robustness of the application by ensuring that any errors in the relationship are handled properly.
Updating Information in the Pivot Table
Often, we need to update data in the pivot table without creating a new relationship. For this, we use updateExistingPivot()
:
$user->projects()->updateExistingPivot($projectId, ['role' => 'supervisor']);
This method only updates the specified fields in the existing relationship. Note: It does not create a new relationship if it does not exist, which differentiates it from other methods.
Filtering Relationships by Pivot Data
It is possible to filter relationships based on data from the pivot table. A common example is retrieving all projects where a user has a specific role.
$user->projects()->wherePivot('role', 'admin')->get();
When using withPivot()
, we can also access this data when querying.
Validating Data Before Saving
It is essential to validate the data before saving it in the pivot table. Laravel allows us to perform validations using the $rules
attribute in controllers:
$request->validate([
'role' => 'required|string',
'start_date' => 'required|date'
]);
Maintaining Data Consistency
To maintain data integrity, it is important to ensure that the pivot table is properly structured and that relationships are only created when the related models exist. Using foreign keys and referential integrity rules helps ensure data consistency and avoid inconsistencies.
Conclusion
Laravel 11, with Eloquent, simplifies managing many-to-many relationships, including using pivot tables with additional data. It is essential to understand the differences between sync()
and attach()
, how to use withPivot()
correctly, and how to validate and handle exceptions to build a robust application. With these techniques, you can efficiently and safely manage complex relationships.