10 Architecture Principles That Will Transform Your Laravel Development
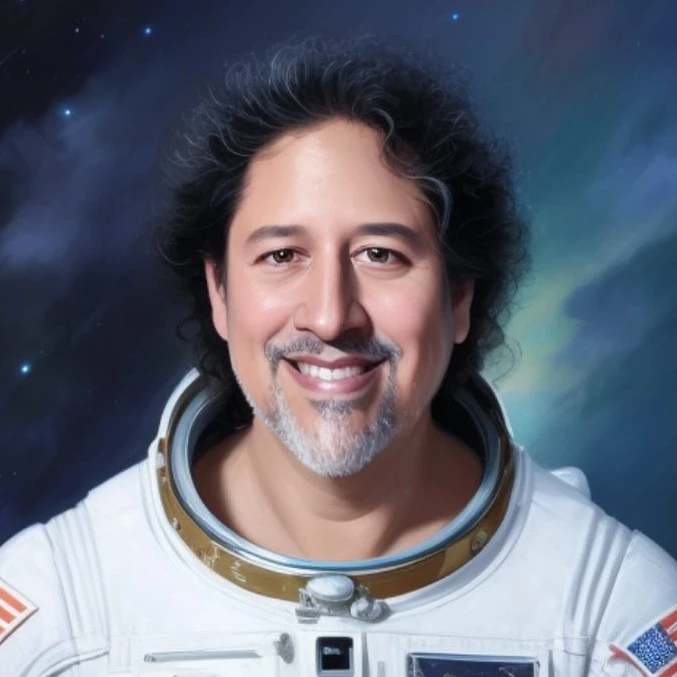
Herminio Heredia
3 months ago
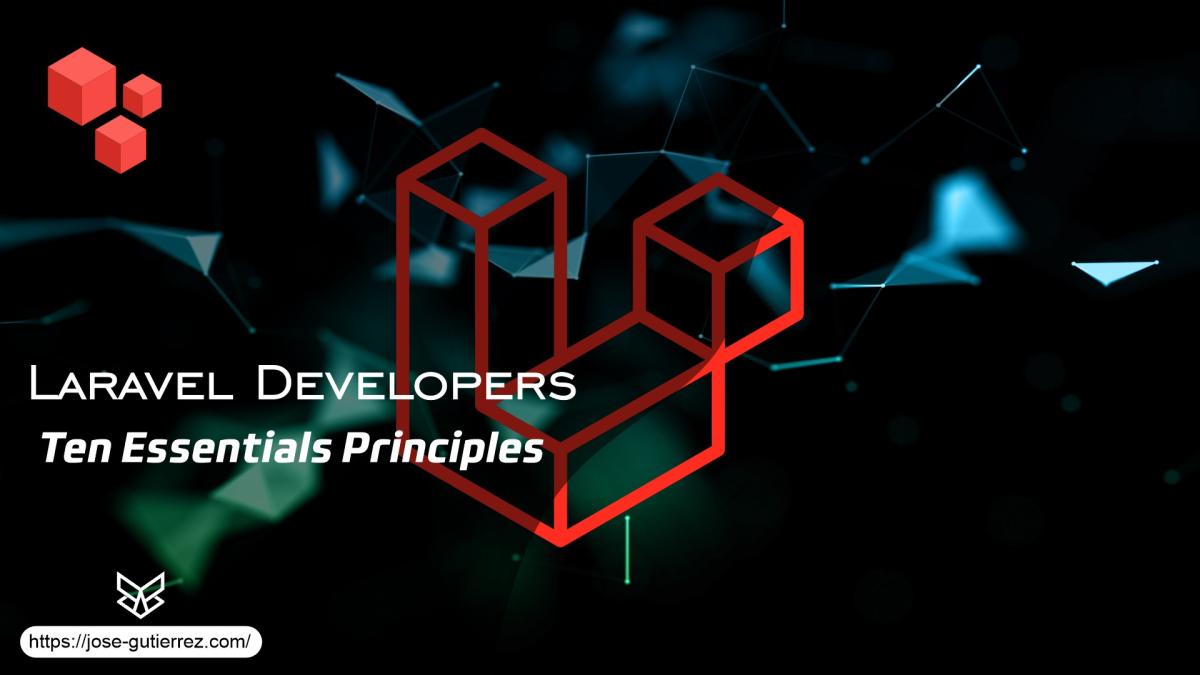
Worried about long-term scalability?
Is maintenance becoming a nightmare?
Relax! You’re not alone.
Many developers, even those with Laravel experience, face these challenges. But the good news is there’s a solution: mastering software architecture principles.
Software architecture, often seen as an abstract concept, is the backbone of any successful application. Think of it like a building’s blueprint. Without a good blueprint, the building could be unstable, hard to modify, and ultimately prone to collapse. The same goes for your code.
In this article, we’ll dive into 10 software architecture principles that, if applied correctly, will transform the way you develop with Laravel. Not only that, they will give you the confidence to build high-impact, scalable, and easy-to-maintain applications. Get ready for a journey that will elevate your level as a Laravel developer. Let’s get started!
1. SRP: The Single Responsibility Principle
Why should your code have one reason to change?
Imagine you have a Swiss Army knife. It’s great for many things, right? But what if you need a really good screwdriver? You’ll probably turn to a specialized tool. The Single Responsibility Principle (SRP) follows that same logic.
Essentially, the SRP states that a class (or module, or function) should have one, and only one, reason to change.
How does it apply to Laravel?
Let’s look at a classic example:
// Bad! ❌
class User {
public function register($data) {
// 1. Validate data
// 2. Create the user in the database
// 3. Send a welcome email
// 4. Log the registration
}
}
This User
class is doing too many things. It has multiple responsibilities. If the email-sending logic changes, you have to modify User
. If the logging method changes, guess what? You have to modify User
again!
The solution: Separate responsibilities.
// Good! ✅
class User {
public function register($data) {
// 1. Validate data (could go in a Form Request)
// 2. Create the user in the database
}
}
class UserRegistrationService {
public function handle($user, $data) {
$user->register($data);
$this->sendWelcomeEmail($user);
$this->logRegistration($user);
}
protected function sendWelcomeEmail($user) {
// Logic to send the welcome email
}
protected function logRegistration($user) {
// Logic to log the registration
}
}
Now we have a User
class focused on handling user-related tasks, and a UserRegistrationService
class that orchestrates the actions, separating responsibilities and reducing coupling. You could also use the Command pattern to encapsulate each operation.
Benefits of SRP in Laravel:
- Cleaner, more readable code: Smaller, more focused classes.
- Easier maintenance: Changes in one responsibility don’t affect others.
- Better testability: Easier to test classes with a single responsibility.
- Greater reusability: You can reuse smaller components in different parts of your application.
2. OCP: The Open/Closed Principle
Extend your code, don’t modify it.
The Open/Closed Principle (OCP) is one of the pillars of robust design. This principle tells us:
“Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.”
What does this mean in practical terms? It means you should be able to add new functionalities to a class without changing its existing code. Sounds contradictory, right? But this is where interfaces and abstract classes come into play.
Example in Laravel: Flexible notifications
Let’s say you have a notification system in your Laravel application. Initially, you only send notifications via email:
// Bad! ❌
class NotificationService {
public function send($user, $message) {
// Logic to send an email
Mail::to($user->email)->send(new NotificationMail($message));
}
}
What if you then want to add support for SMS or Slack notifications? You’d have to modify the NotificationService
class, violating OCP.
Applying OCP:
// Good! ✅
interface NotificationChannel {
public function send($user, $message);
}
class EmailChannel implements NotificationChannel {
public function send($user, $message) {
Mail::to($user->email)->send(new NotificationMail($message));
}
}
class SMSChannel implements NotificationChannel {
public function send($user, $message) {
// Logic to send SMS
}
}
class SlackChannel implements NotificationChannel {
public function send($user, $message) {
// Logic to send Slack message
}
}
class NotificationService {
protected $channel;
public function __construct(NotificationChannel $channel) {
$this->channel = $channel;
}
public function send($user, $message) {
$this->channel->send($user, $message);
}
}
// Usage:
$emailService = new NotificationService(new EmailChannel());
$emailService->send($user, "Hello!");
$smsService = new NotificationService(new SMSChannel());
$smsService->send($user, "Hello!");
Now, NotificationService
is closed to modification but open to extension. You can add new notification channels (Slack, Telegram, etc.) simply by creating new classes that implement the NotificationChannel
interface, without touching the NotificationService
code.
Benefits of OCP in Laravel:
- Reduced risk of introducing bugs: You don’t change existing code that already works.
- Greater flexibility: Easily add new functionalities.
- More maintainable code: Code that follows OCP is easier to understand and maintain.
- Loose coupling: Classes depend on abstractions.
3. LSP: The Liskov Substitution Principle
If it looks like a duck and swims like a duck…
The Liskov Substitution Principle (LSP) is a bit more subtle than the previous ones, but just as important. Simply put, LSP states:
“If you have a base class and a derived class, you should be able to use the derived class anywhere the base class is used without altering the correct functioning of the program.”
In other words, subclasses should be substitutable for their base classes.
Example in Laravel: Geometric shapes
// Bad! ❌
class Rectangle {
protected $width;
protected $height;
public function setWidth($width) {
$this->width = $width;
}
public function setHeight($height) {
$this->height = $height;
}
public function getArea() {
return $this->width * $this->height;
}
}
class Square extends Rectangle {
public function setWidth($width) {
$this->width = $width;
$this->height = $width; // Problem!
}
public function setHeight($height) {
$this->height = $height;
$this->width = $height; // Problem!
}
}
// Usage:
$rectangle = new Rectangle();
$rectangle->setWidth(5);
$rectangle->setHeight(10);
echo $rectangle->getArea(); // 50
$square = new Square();
$square->setWidth(5);
$square->setHeight(10); // A square can’t have different width and height!
echo $square->getArea(); // 100, which is incorrect!
In this example, Square
extends Rectangle
but violates LSP. If you try to use a Square
as if it were a Rectangle
(setting different widths and heights), the behavior changes and the area calculation breaks.
Applying LSP: A better way is to use an interface:
interface Shape {
public function getArea();
}
class Rectangle implements Shape {
protected $width;
protected $height;
public function setWidth($width) {
$this->width = $width;
}
public function setHeight($height) {
$this->height = $height;
}
public function getArea() {
return $this->width * $this->height;
}
}
class Square implements Shape {
protected $side;
public function setSide($side) {
$this->side = $side;
}
public function getArea() {
return $this->side * $this->side;
}
}
Now, both classes implement an interface that defines the contract.
Benefits of LSP in Laravel:
- More predictable code: You know you can substitute subclasses without surprises.
- Higher robustness: Avoid subtle bugs caused by LSP violations.
- Better design: Fosters a well-structured class hierarchy.
- Facilitates polymorphism.
4. ISP: The Interface Segregation Principle
No “fat” interfaces, please.
The Interface Segregation Principle (ISP) tells us:
“No client should be forced to depend on methods it does not use.”
In other words, it’s better to have many small, specific interfaces than one “fat” interface that does everything. This prevents classes from having to implement methods they don’t need.
Example in Laravel: Workers and machines
// Bad! ❌
interface Worker {
public function work();
public function eat();
public function sleep();
public function operateMachine();
}
class HumanWorker implements Worker {
// Implements work(), eat(), sleep()
// But what if this worker doesn’t operate machines?
public function work(){...}
public function eat(){...}
public function sleep(){...}
public function operateMachine(){
// Shouldn’t be here
}
}
class RobotWorker implements Worker {
// Implements work(), operateMachine()
// But do robots eat or sleep?
public function work(){...}
public function eat(){ // Shouldn’t be here }...}
public function sleep(){ // Shouldn’t be here }...}
public function operateMachine(){...}
}
The Worker
interface is too broad. It forces classes to implement methods that are not always relevant.
Applying ISP:
// Good! ✅
interface Workable {
public function work();
}
interface Eatable {
public function eat();
}
interface Sleepable {
public function sleep();
}
interface MachineOperable {
public function operateMachine();
}
class HumanWorker implements Workable, Eatable, Sleepable {
public function work(){...}
public function eat(){...}
public function sleep(){...}
}
class RobotWorker implements Workable, MachineOperable {
public function work(){...}
public function operateMachine(){...}
}
class SuperHuman implements Workable, Eatable, Sleepable, MachineOperable {
public function work(){...}
public function eat(){...}
public function sleep(){...}
public function operateMachine(){...}
}
Now we have smaller, more specific interfaces. Each class only implements the interfaces it actually needs, avoiding unnecessary methods.
Benefits of ISP in Laravel:
- More cohesive interfaces: Each interface has a clear purpose.
- Reduced coupling: Classes do not depend on interfaces they don’t use.
- More flexible code: It’s easier to add new functionality without affecting existing classes.
- Easier to understand and maintain.
5. DIP: The Dependency Inversion Principle
Depend on abstractions, not on concretions.
The Dependency Inversion Principle (DIP) is crucial for building decoupled, flexible applications. It states two key points:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In simpler terms, DIP tells us to depend on interfaces or abstract classes, not on concrete implementations.
Example in Laravel: Reports and formats
// Bad! ❌
class ReportGenerator {
protected $data;
public function __construct($data){
$this->data = $data;
}
public function generatePDF() {
// Specific logic to generate a PDF
$pdf = new PDFGenerator($this->data); // Direct dependency on PDFGenerator
return $pdf->generate();
}
}
ReportGenerator
directly depends on PDFGenerator
. If you want to add support for other formats (CSV, Excel, etc.), you’d have to modify ReportGenerator
, violating OCP (yet again!).
Applying DIP (and Dependency Injection):
// Good! ✅
interface ReportGenerator {
public function generate();
}
class PDFGenerator implements ReportGenerator {
protected $data;
public function __construct($data){
$this->data = $data;
}
public function generate() {
// Logic to generate PDF
}
}
class CSVGenerator implements ReportGenerator {
protected $data;
public function __construct($data){
$this->data = $data;
}
public function generate() {
// Logic to generate CSV
}
}
class ReportService {
protected $generator;
public function __construct(ReportGenerator $generator) {
$this->generator = $generator; // Dependency Injection
}
public function generateReport() {
return $this->generator->generate();
}
}
// Usage:
$reportData = [...];
$pdfService = new ReportService(new PDFGenerator($reportData));
$pdfReport = $pdfService->generateReport();
$csvService = new ReportService(new CSVGenerator($reportData));
$csvReport = $csvService->generateReport();
Now, ReportService
depends on the abstraction ReportGenerator
rather than a concrete implementation, and it uses Dependency Injection. You can use any generator that implements this interface without modifying ReportService
. Laravel makes Dependency Injection easy through its Service Container.
Benefits of DIP in Laravel:
- Decoupling: Classes are not tightly coupled to concrete implementations.
- Greater flexibility: You can switch implementations without affecting other classes.
- Better testability: You can use mocks or stubs of dependencies for unit testing.
- More maintainable code: Easier to understand and modify.
- Facilitates component reuse.
6. DRY: Don’t Repeat Yourself
Laziness as a programmer’s virtue.
The DRY principle (“Don’t Repeat Yourself”) is one of the most basic yet powerful concepts in software development. Its message is clear:
Avoid code duplication whenever possible.
Each piece of knowledge (logic, configuration, data) should have a single authoritative representation within the system.
Why is it so important to avoid duplication?
- Maintenance: If you have the same logic in multiple places, any change requires updating all those places. This increases the risk of bugs and makes maintenance a nightmare.
- Consistency: Duplication leads to inconsistencies. If you forget to update one of the copies, you’ll have different behaviors in different parts of the application.
- Readability: Duplicated code is harder to read and understand.
- Code size: Duplication unnecessarily inflates the size of your codebase.
Examples of duplication in Laravel (and how to avoid it):
- Repeated validation logic: Instead of repeating the same validation in multiple controllers or Form Requests, create custom validation rules or use traits.
- Repeated Eloquent queries: Define local or global scopes in your models to encapsulate common queries.
- Repeated view fragments: Use partials, Blade components, or layouts to reuse HTML sections.
-
Duplicated configuration: Use Laravel’s configuration files (
config/
) and environment variables (.env
) to centralize settings. - Repeated business logic: Use services, repositories, actions, or the Command pattern.
Example: Eloquent Scopes
// Bad! ❌
// In several controllers...
$activeUsers = User::where('status', 'active')->get();
$inactiveUsers = User::where('status', 'inactive')->get();
// Good! ✅
// In the User model
class User extends Model {
public function scopeActive($query) {
return $query->where('status', 'active');
}
public function scopeInactive($query) {
return $query->where('status', 'inactive');
}
}
// In controllers...
$activeUsers = User::active()->get();
$inactiveUsers = User::inactive()->get();
Benefits of DRY in Laravel:
- Cleaner, more concise code.
- Much easier maintenance.
- Lower risk of bugs.
- Greater consistency across the application.
7. KISS: Keep It Simple, Stupid
The elegance of simplicity.
KISS (“Keep It Simple, Stupid”) is a call for simplicity in software design and implementation. It’s not about being “stupid” but about avoiding unnecessary complexity.
“Complexity is the enemy of execution.” — Tony Robbins
Why is simplicity so important?
- Readability: Simple code is easier to understand, both for you and for other developers (including your future self!).
- Maintenance: Simple code is easier to maintain and modify.
- Debugging: It’s easier to find and fix errors in simple code.
- Performance: Often, simpler code is more efficient.
- Fewer bugs: Complexity increases the probability of errors.
How to apply KISS in Laravel:
- Use the framework’s tools: Laravel offers many built-in features (Eloquent, Blade, Collections, etc.). Take advantage of them instead of reinventing the wheel.
- Avoid over-engineering: Don’t add layers of abstraction or design patterns just because they look cool.
- Break down large problems into smaller ones: Use smaller, more focused classes, methods, and functions.
- Use descriptive names: Use clear, meaningful names for variables, methods, classes, etc.
- Comment the code, but not excessively: Comments should explain why something is done, not what is being done (the code itself should be clear enough for that).
- Refactor regularly: As your code evolves, look for opportunities to simplify it.
Example: Avoiding over-engineering
// Bad! ❌ (Over-engineering)
interface DataFetcher {
public function fetch();
}
class DatabaseDataFetcher implements DataFetcher {
public function fetch() {
return DB::table('users')->get();
}
}
class DataProcessor {
protected $fetcher;
public function __construct(DataFetcher $fetcher)
{
$this->fetcher = $fetcher;
}
public function process() {
$data = $this->fetcher->fetch();
// Process the data...
}
}
// Usage
$processor = new DataProcessor(new DatabaseDataFetcher());
$processor->process();
// Good! ✅ (Simpler)
class UserService {
public function getUsers() {
return DB::table('users')->get(); // Directly, no unnecessary layers
}
}
// Usage
$service = new UserService();
$users = $service->getUsers();
In this case, the “simple” version is more direct and easier to understand. The version with interfaces and additional classes adds complexity without clear benefit in this particular context.
Benefits of KISS in Laravel:
- Faster development.
- Easier-to-understand, maintainable code.
- Fewer bugs.
- Often better performance.
8. YAGNI: You Ain’t Gonna Need It
The art of not doing.
YAGNI (“You Ain’t Gonna Need It”) is a principle that encourages you to resist the temptation to add features you might need in the future but do not need now.
“The fastest way to get something done is to not do it.” — Gerry Weinberg
Why is YAGNI important?
- Saves time and effort: You avoid developing code that will never be used.
- Simpler code: Less code means less complexity.
- Fewer bugs: Less code also means fewer opportunities for errors.
- Greater flexibility: It’s easier to pivot if you don’t have a large codebase with unused features.
How to apply YAGNI in Laravel:
- Start with the minimum viable product: Implement only the functionalities that are absolutely necessary for the current version.
- Resist the urge to “plan for the future”: Don’t add code “just in case.”
- Rely on refactoring: If you need a feature in the future, you can always add it later.
- Use version control: Git allows you to experiment with new features without fear of breaking existing code.
- Focus on actual user needs: Concentrate on what users really need, not on what you think they might need.
Example: Not adding features “just in case”
Suppose you’re building a simple blog. You might be tempted to add features like:
- Nested comments
- Multiple categories per post
- Tag system
- Voting system
- Advanced WYSIWYG editor
But do you really need all those features right now? Probably not. Start with the basics (publishing articles) and add new features only when they’re truly required.
Benefits of YAGNI in Laravel:
- Faster, more efficient development.
- Cleaner, simpler codebase.
- Less maintenance.
- Greater focus on real user needs.
9. The Law of Demeter (LoD)
Talk only to your close friends.
The Law of Demeter, also known as the Principle of Least Knowledge, aims to reduce coupling between classes. Essentially, it states:
An object should only interact with its “close friends.”
This means that a method of an object should only call methods of:
- The object itself.
- Objects passed as parameters to the method.
- Objects created within the method.
- Objects that are direct attributes of the object.
Why is the Law of Demeter important?
- Reduced coupling: Classes depend less on each other.
- Improved encapsulation: A class’s internal details stay hidden.
- Easier maintenance: Changes in one class are less likely to affect other classes.
- More readable code: It’s easier to understand interactions between objects.
Example in Laravel: Avoiding a “train wreck” of calls
// Bad! ❌ (Violation of the Law of Demeter)
class Order {
protected $customer;
// constructor and so on
public function getCustomer(){
return $this->customer;
}
}
class Customer {
protected $address;
// constructor and so on
public function getAddress(){
return $this->address;
}
}
class Address {
protected $city;
// constructor and so on
public function getCity(){
return $this->city;
}
}
// In some controller...
$order = new Order();
$city = $order->getCustomer()->getAddress()->getCity(); // "Train wreck" of calls!
This chain of calls ($order->getCustomer()->getAddress()->getCity()
) violates the Law of Demeter. The controller needs to “know” the internal structure of Order
, Customer
, and Address
.
Applying the Law of Demeter:
// In Order
class Order {
// ...
public function getCustomerCity() {
return $this->customer->getAddressCity(); // Delegation
}
}
// In Customer
class Customer {
// ...
public function getAddressCity() {
return $this->address->getCity();
}
}
// In the controller...
$order = new Order();
$city = $order->getCustomerCity(); // Much better
Now, Order
delegates the responsibility of getting the city to Customer
, which in turn delegates it to Address
. The controller only interacts with Order
, its “close friend.”
Benefits of the Law of Demeter in Laravel:
- More decoupled, flexible code.
- Better encapsulation.
- Easier maintenance.
- More readable code.
10. Composition over Inheritance
Prefer “has-a” to “is-a.”
Inheritance is a powerful tool in object-oriented programming, but it’s often overused. “Composition over inheritance” encourages us to prefer composition (an object has another object) rather than inheritance (an object is another object).
Why is composition often better than inheritance?
- Greater flexibility: Composition lets you change an object’s behavior at runtime, while inheritance is static.
- Looser coupling: Composition creates weaker relationships between classes than inheritance does.
- Avoid “base class fragility”: Changes in a base class can cascade and affect all subclasses.
- Better reuse: It’s easier to reuse smaller, specific components than large, complex base classes.
- Avoid multiple inheritance issues (which doesn’t exist in PHP but can be emulated with traits, sometimes leading to collisions).
Example in Laravel: A video game “character”
// Bad! ❌ (Inheritance)
class Character {
// ...
}
class Warrior extends Character {
public function attack() {
// Sword attack
}
}
class Mage extends Character {
public function attack() {
// Magic attack
}
}
// What about a magic warrior?
class MagicWarrior extends Warrior // or Mage? Problem!
{
public function attack() {
// ???
}
}
Inheritance becomes problematic when you need to combine behaviors.
Applying Composition:
// Good! ✅ (Composition)
interface AttackBehavior {
public function attack();
}
class SwordAttack implements AttackBehavior {
public function attack() {
// Sword attack logic
}
}
class MagicAttack implements AttackBehavior {
public function attack() {
// Magic attack logic
}
}
class Character {
protected $attackBehavior;
public function __construct(AttackBehavior $attackBehavior) {
$this->attackBehavior = $attackBehavior;
}
public function attack() {
$this->attackBehavior->attack();
}
}
// Usage:
$warrior = new Character(new SwordAttack());
$warrior->attack(); // Sword attack
$mage = new Character(new MagicAttack());
$mage->attack(); // Magic attack
$magicWarrior = new Character(new MagicAttack()); // Easy to create!
$magicWarrior->attack();
// Or even combine
class CombineAttack implements AttackBehavior {
protected $attackBehaviors = [];
public function addAttack(AttackBehavior $attackBehavior)
{
$this->attackBehaviors[] = $attackBehavior;
}
public function attack()
{
foreach ($this->attackBehaviors as $attack) {
$attack->attack();
}
}
}
$combineWarrior = new Character(new CombineAttack());
$combineWarrior->attackBehavior->addAttack(new SwordAttack());
$combineWarrior->attackBehavior->addAttack(new MagicAttack());
$combineWarrior->attack(); // Both sword and magic attacks
Now, a Character
has an AttackBehavior
. You can create different types of characters simply by combining different behaviors.
Benefits of Composition in Laravel:
- More flexible, adaptable code.
- Reduced coupling.
- Better code reuse.
- Avoid multiple inheritance problems.
Conclusion: Transform Your Laravel Development
We’ve covered a lot of ground, exploring 10 software architecture principles that can transform how you develop with Laravel. From single responsibility to composition over inheritance, these principles will guide you toward cleaner, more robust, scalable, and maintainable code.
Remember that software architecture is not a magic formula, but a set of principles you should adapt to your specific needs. It’s not about applying all principles strictly in every situation, but about understanding them and using them as tools to make better design decisions.
Start applying these principles today. There’s no need for a radical overnight change. Begin with small steps, refactoring your existing code and applying these principles in your new projects.
Over time, you’ll notice a big difference in your code quality and in your productivity as a developer. And most importantly, you’ll enjoy the development process more!
This journey of learning doesn’t end here. Keep exploring, experimenting, and sharing your knowledge with the community. The world of software development is constantly evolving, and there is always something new to learn!
So, go for it! Take charge of your Laravel development and build amazing applications. The power is in your hands!