Laravel & Vue: Real-Time Notifications with Reverb, Redis, and TDD - 01
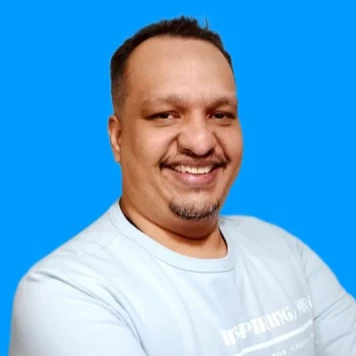
José Rafael Gutierrez
2 months ago
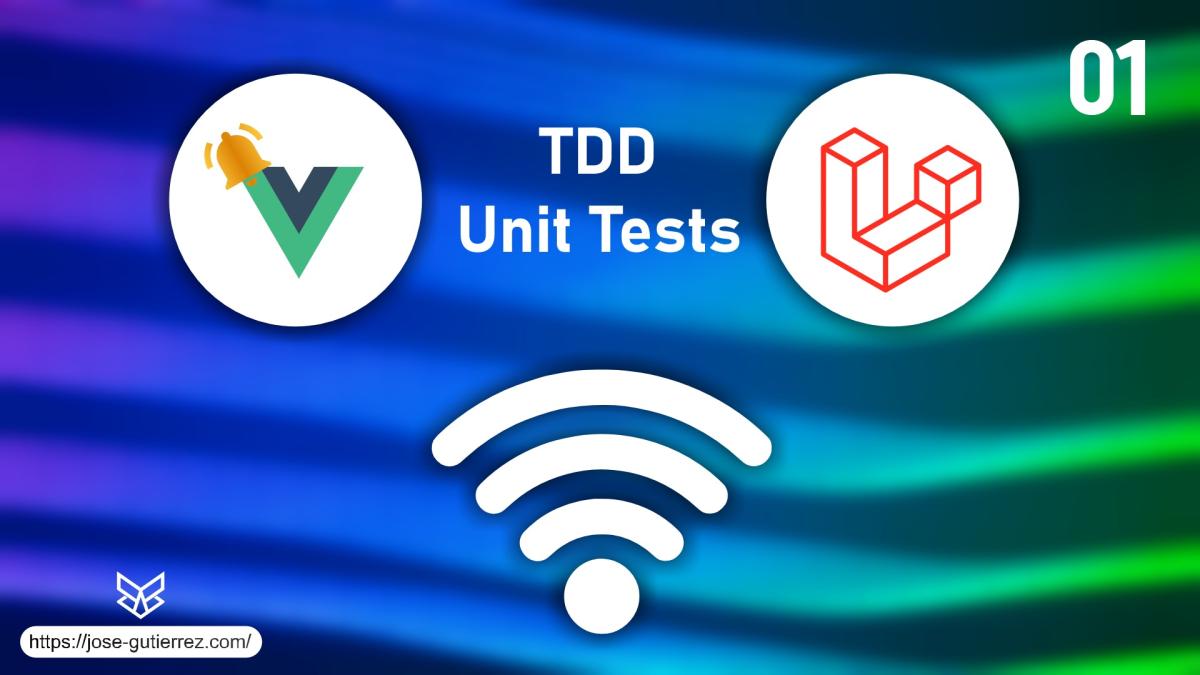
Chapter 1: Introduction and Configuration of Reverb and Redis in a Laravel Environment
Objective
Configure Reverb in a Laravel project to enable real-time notifications, integrating Redis as a caching system and validating a basic notification model using TDD (Test-Driven Development).
Introduction
Reverb is a native Laravel solution for real-time broadcasting that simplifies WebSocket usage without relying on third-party services. Combined with Redis, it allows for efficient notification management. This chapter lays the foundation of the system, following the red → green → refactor cycle of TDD.
Initial Setup
Create the Laravel Project
1. Generate a new Laravel project:
composer create-project laravel/laravel shipping-notifications
cd shipping-notifications
2. Install Sail for Docker-based environments:
composer require laravel/sail --dev
php artisan sail:install
./vendor/bin/sail up -d
3. Verify that Laravel is running by visiting http://localhost.
Installing and Configuring Reverb
Add Reverb
1. Install Reverb in your project:
./vendor/bin/sail artisan install:broadcasting
2. During installation:
- Select Reverb as the driver.
- Configure
localhost
as the host,8080
as the port, andhttp
as the scheme.
3. Update your .env
file with these values:
BROADCAST_CONNECTION=reverb
QUEUE_CONNECTION=redis
Reverb-specific variables will be automatically added to .env
during the setup. You’ll see something like this:
REVERB_APP_ID=155758
REVERB_APP_KEY=0h6i5q1g0ymdolgci5mt
REVERB_APP_SECRET=d9rqhmvvjyaxp4kakgj9
REVERB_HOST="localhost"
REVERB_PORT=8080
REVERB_SCHEME=http
Configuring Redis
1. Ensure Redis is correctly set up in .env
:
CACHE_STORE=redis
QUEUE_CONNECTION=redis
REDIS_HOST=redis
REDIS_PORT=6379
2. Test Redis with Tinker:
./vendor/bin/sail artisan tinker
Inside Tinker:
Cache::put('key', 'value', 10);
Cache::get('key'); // Returns 'value'
Configuring Tests
Modify the phpunit.xml
file to use an in-memory SQLite database during tests:
<php>
<env name="DB_CONNECTION" value="sqlite"/>
<env name="DB_DATABASE" value=":memory:"/>
</php>
Implementing the Notification Model
1. Generate the model with its migration:
./vendor/bin/sail artisan make:model Notification -m
2. Define the table structure in the migration:
Schema::create('notifications', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained()->cascadeOnDelete();
$table->string('status');
$table->timestamps();
});
3. Run the migrations:
./vendor/bin/sail artisan migrate
Test-Driven Development (TDD)
Writing the Initial Test
Create a test in tests/Feature/NotificationTest.php
:
namespace Tests\Feature;
use App\Models\Notification;
use App\Models\User;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class NotificationTest extends TestCase
{
use RefreshDatabase;
public function test_it_creates_a_notification()
{
// Given
$user = User::factory()->create();
$data = [
'user_id' => $user->id,
'status' => 'Sent',
];
// When
Notification::create($data);
// Then
$this->assertDatabaseHas('notifications', $data);
}
}
Test Breakdown
- GIVEN: We set up the environment for the test by creating a user and defining the notification data.
- WHEN: We execute the action under test, in this case, creating a notification.
-
THEN: We verify that the data has been saved correctly in the database using
assertDatabaseHas
.
Executing the Red → Green → Refactor Cycle
1. Red: Run the initial test:
./vendor/bin/sail artisan test
It will fail because the model hasn't been implemented yet.
2. Green: Implement the model in app/Models/Notification.php
:
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Notification extends Model
{
use HasFactory;
protected $fillable = ['user_id', 'status'];
}
Run the test again. It should now pass:
./vendor/bin/sail artisan test
3. Refactor: Review and refine your code if necessary. For instance, ensure proper validation for the notification data in future stages.
Tip: Create an Alias for sail command
To simplify the use of ./vendor/bin/sail
, you can create an alias as follows:
For Bash
Add this line to the ~/.bashrc
file:
alias sail='./vendor/bin/sail'
Then, reload the configuration file with:
source ~/.bashrc
For Zsh
Add this line to the ~/.zshrc
file:
alias sail='./vendor/bin/sail'
Then, reload the configuration file with:
source ~/.zshrc
With this, you can use the sail
command instead of ./vendor/bin/sail
.
Conclusion
In this chapter, we configured Reverb and Redis, created a basic notification model, and validated its functionality using TDD. In the next chapter, we’ll focus on broadcasting real-time notifications using Reverb.
Keep going! 🚀